Top 10 Java Serialization Interview questions
Java Serialization is one of important concept but it’s been rarely used as persistence solution and developer mostly overlooked java serialization API. As per my experience Java Serialization is quite an important topic in any java interview, In almost all the interview I have faced there is one or two java serialization questions and I have seen interview where after few question on serialization candidate start feeling uncomfortable because of lack of experience in this area. They don’t know how to serialize object in java or they are not familiar with any java serialization example to explain.
Top 10 Java Serialization Interview questions
Java serialization process only continues in object hierarchy till the class is Serializable i.e. implements Serializable interface in Java And values of the instance variables inherited from super class will be initialized by calling constructor of Non-Serializable Super class during deserialization process . once the constructor chaining will started it wouldn't be possible to stop that , hence even if classes higher in hierarchy implements Serializable interface , there constructor will be executed.
Top 10 Java Serialization Interview questions
Most commercial project uses either database or memory mapped file or simply flat file for there persistence requirement and only few of them rely on java serialization. Anyway this post is not a java serialization tutorial or how to serialize in java but about few interview questions around java serialization which is worth to have a look before going for any java interview and surprising yourself with some unknown contents. for those who are not familiar about java Serialization "Java serialization is the process which is used to serialize object in java by storing object’s state into a file with extension .ser and recreating object's state from that file, this reverse process is called deserialization.
The Java Serialization API provides a standard mechanism for developers to handle object serialization using Serializable and Externalizable interface.
This article is in continuation of my previous article Top 20 Core Java Interview Question and 10 Interview questions on Singleton Pattern in Java So here we go.
1) What is the difference between Serializable and Externalizable interface in Java?
This is most frequently asked question in java serialization interview. Here is my version Externalizable provides us writeExternal () and readExternal () method which gives us flexibility to control java serialization mechanism instead of relying on java's default serialization. Correct implementation of Externalizable interface can improve performance of application drastically.
2) How many methods Serializable has? If no method then what is the purpose of Serializable interface?
Serializable interface exists in java.io package and forms core of java serialization mechanism. It doesn't have any method and also called Marker Interface. When your class implements Serializable interface it becomes Serializable in Java and gives compiler an indication that use Java Serialization mechanism to serialize this object.
3) What is serialVersionUID? What would happen if you don't define this?
SerialVersionUID is an ID which is stamped on object when it get serialized usually hashcode of object, you can use tool serialver to see serialVersionUID of a serialized object . serialVersionUID is used for version control of object. you can specify serialVersionUID in your class file also. Consequence of not specifying serialVersionUID is that when you add or modify any field in class then already serialized class will not be able to recover because serialVersionUID generated for new class and for old serialized object will be different. Java serialization process relies on correct serialVersionUID for recovering state of serialized object and throws
java.io.InvalidClassException in case of
serialVersionUID mismatch.
4) While serializing you want some of the members not to serialize? How do you achieve it?
this is sometime also asked as what is the use of transient variable, does transient and static variable getsserialized or not etc. so if you don't want any field to be part of object's state then declare it either static or transient based on your need and it will not be included during java serialization process.
5) What will happen if one of the members in the class doesn't implement Serializable interface?
If you try to serialize an object of a class which implements Serializable, but the object includes a reference to an non- Serializable class then a ‘NotSerializableException’ will be thrown at runtime and this is why I always put a SerializableAlert (comment section in my code) to instruct developer to remember this fact while adding a new field in a Serializable class.
6) If a class is Serializable but its super class in not, what will be the state of the instance variables inherited from super class after deserialization?
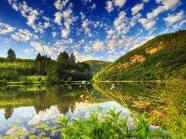
7) Can you Customize Serialization process or can you override default Serialization process in Java?
The answer is yes you can. We all know that for serializing an object objectOutputStream.writeObject (saveThisobject) is invoked and for reading object ObjectInputStream.readObject () is invoked but there is one more thing which Java Virtual Machine provides you is to define these two method in your class. If you define these two methods in your class then JVM will invoke these two methods instead of applying default serialization mechanism. You can customize behavior of object serialization or deserialization here by doing any kind of pre or post processing task. Important point to note is making these methods private to avoid being inherited, overridden or overloaded. Since only Java Virtual Machine can call private method integrity of your class will remain and Java Serialization will work as normal.
8) Suppose super class of a new class implement Serializable interface, how can you avoid new class to being serialized?
If Super Class of a Class already implements Serializable interface in Java then its already serializable in Java, since you can not unimplemented an interface its not really possible to make it Non Serializable class but yes there is a way to avoid serialization of new class. To avoid java serialization you need to implement writeObject () and readObject () method in your Class and need to throw NotSerializableException from those method. This is another benefit of customizing java serialization process as described in above question and normally it asked as follow-up question as interview progresses.
9) Which methods are used during Serialization and DeSerialization process in java?
This is quite a common question basically interviewer is trying to know that whether you are familiar with usage of readObject (), writeObject (), readExternal () and writeExternal () or not. Java Serialization is done by java.io.ObjectOutputStream class. That class is a filter stream which is wrapped around a lower-level byte stream to handle the serialization mechanism. To store any object via serialization mechanism we call objectOutputStream.writeObject (saveThisobject) and to deserialize that object we call ObjectInputStream.readObject () method. Call to writeObject () method trigger serialization process in java. one important thing to note about readObject() method is that it is used to read bytes from the persistence and to create object from those bytes and its return an Object which needs to be casted on correct type.
10) Suppose you have a class which you serialized it and stored in persistence and later modified that class to add a new field. What will happen if you deserialize the object already serialized?
It depends on whether class has its own serialVersionUID or not. As we know from above question that if we don't provide serialVersionUID in our code java compiler will generate it and normally it’s equal to hash code of object. by adding any new field there is chance that new serialVersionUID generated for that class version is not the same of already serialized object and in this case Java Serialization API will throwjava.io.InvalidClassException and this is the reason its recommended to have your own serialVersionUID in code and make sure to keep it same always for a single class.
11) What are the compatible changes and incompatible changes in Java Serialization Mechanism?
The real challenge lies with change in class structure by adding any field, method or removing any field or method is that with already serialized object. As per Java Serialization specification adding any field or method comes under compatible change and changing class hierarchy or unimplementing Serializable interfaces some under non compatible changes. For complete list of compatible and non compatible changes I would advise reading java serialization specification.
12) Can we transfer a Serialized object vie network?
Yes you can transfer a Serialized object via network because java serialized object remains in form of bytes which can be transmitter via network.
13) Which kind of variables is not serialized during Java Serialization?
This question asked sometime differently but the purpose is same whether Java developer knows specifics aboutstatic and transient variable or not. Since static variables belong to the class and not to an object they are not the part of the state of object so they are not saved during Java Serialization process. As Java Serialization only persist state of object and not object itself. Transient variables are also not included in java serialization process and are not the part of the object’s serialized state. After this question sometime interviewer ask a follow-up if you don't store values of these variables then what would be value of these variable once you deserialize and recreate those object? This is for you guys to think about :)
Please tweet or submit to reddit if your like this article
2 comments:
adidas stan smith women
air jordan
cheap air jordan
ray ban sunglasses outlet
adidas nmd
nike huarache sale
michael kors outlet online
tiffany jewelry
kobe shoes
adidas nmd
michael kors outlet
yeezy boost 350
chrome hearts
http://www.cheapairjordan.us
nike zoom kobe
tiffany and co outlet online
kobe basketball shoes
cheap tiffanys
tiffany and co outlet
adidas tubular
christian louboutin outlet
kobe 9
supreme clothing
balenciaga shoes
balenciaga trainers
golden goose
mbt
100% real jordans for cheap
supreme clothing
michael kors handbags
balenciaga shoes
Post a Comment