How hibernate second level cache works?
Caching is facility provided by ORM frameworks which help users to get fast running web application, while help framework itself to reduce number of queries made to database in a single transaction. Hibernate also provide this caching functionality, in two layers.
- Fist level cache: This is enabled by default and works in session scope. Read more about hibernate first level cache.
- Second level cache: This is apart from first level cache which is available to be used globally in session factory scope.
Above statement means, second level cache is created in session factory scope and is available to be used in all sessions which are created using that particular session factory.
It also means that once session factory is closed, all cache associated with it die and cache manager also closed down.
Further, It also means that if you have two instances of session factory (normally no application does that), you will have two cache managers in your application and while accessing cache stored in physical store, you might get unpredictable results like cache-miss.
It also means that once session factory is closed, all cache associated with it die and cache manager also closed down.
Further, It also means that if you have two instances of session factory (normally no application does that), you will have two cache managers in your application and while accessing cache stored in physical store, you might get unpredictable results like cache-miss.
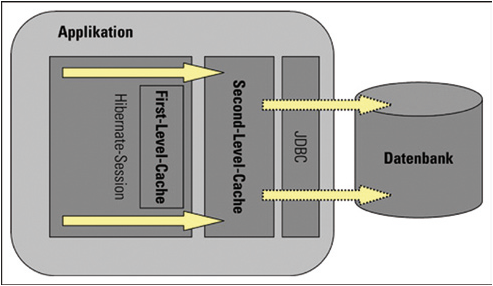
Hibernate first and second level cache
In this tutorial, I am giving concepts around hibernate second level cache and give example using code snippets.
How second level cache works
Lets write all the facts point by point:
- Whenever hibernate session try to load an entity, the very first place it look for cached copy of entity in first level cache (associated with particular hibernate session).
- If cached copy of entity is present in first level cache, it is returned as result of load method.
- If there is no cached entity in first level cache, then second level cache is looked up for cached entity.
- If second level cache has cached entity, it is returned as result of load method. But, before returning the entity, it is stored in first level cache also so that next invocation to load method for entity will return the entity from first level cache itself, and there will not be need to go to second level cache again.
- If entity is not found in first level cache and second level cache also, then database query is executed and entity is stored in both cache levels, before returning as response of load() method.
- Second level cache validate itself for modified entities, if modification has been done through hibernate session APIs.
- If some user or process make changes directly in database, the there is no way that second level cache update itself until “timeToLiveSeconds” duration has passed for that cache region. In this case, it is good idea to invalidate whole cache and let hibernate build its cache once again. You can use below code snippet to invalidate whole hibernate second level cache.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
| /** * Evicts all second level cache hibernate entites. This is generally only * needed when an external application modifies the databaase. */ public void evict2ndLevelCache() { try { Map for (String entityName : classesMetadata.keySet()) { logger.info( "Evicting Entity from 2nd level cache: " + entityName); sessionFactory.evictEntity(entityName); } } catch (Exception e) { logger.logp(Level.SEVERE, "SessionController" , "evict2ndLevelCache" , "Error evicting 2nd level hibernate cache entities: " , e); } } |
To understand more using examples, I wrote an application for testing in which I configured EhCache as 2nd level cache. Lets see various scenarios:
a) Entity is fetched very first time
1
2
3
4
5
6
7
| DepartmentEntity department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); System.out.println(HibernateUtil.getSessionFactory().getStatistics().getEntityFetchCount()); //Prints 1 System.out.println(HibernateUtil.getSessionFactory().getStatistics().getSecondLevelCacheHitCount()); //Prints 0 Output: 1 0 |
Explanation: Entity is not present in either 1st or 2nd level cache so, it is fetched from database.
b) Entity is fetched second time
1
2
3
4
5
6
7
8
9
10
11
12
| //Entity is fecthed very first time DepartmentEntity department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); //fetch the department entity again department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); System.out.println(HibernateUtil.getSessionFactory().getStatistics().getEntityFetchCount()); //Prints 1 System.out.println(HibernateUtil.getSessionFactory().getStatistics().getSecondLevelCacheHitCount()); //Prints 0 Output: 1 0 |
Explanation: Entity is present in first level cache so, it is fetched from there. No need to go to second level cache.
c) Entity is evicted from first level cache and fetched again
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
| //Entity is fecthed very first time DepartmentEntity department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); //fetch the department entity again department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); //Evict from first level cache session.evict(department); department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); System.out.println(HibernateUtil.getSessionFactory().getStatistics().getEntityFetchCount()); //Prints 1 System.out.println(HibernateUtil.getSessionFactory().getStatistics().getSecondLevelCacheHitCount()); //Prints 1 Output: 1 1 |
Explanation: First time entity is fetched from database. Which cause it store in 1st and 2nd level cache. Second load call fetched from first level cache. Then we evicted entity from 1st level cache. So third load() call goes to second level cache and getSecondLevelCacheHitCount() returns 1.
d) Access second level cache from another session
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
| //Entity is fecthed very first time DepartmentEntity department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); //fetch the department entity again department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); //Evict from first level cache session.evict(department); department = (DepartmentEntity) session.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); department = (DepartmentEntity) anotherSession.load(DepartmentEntity. class , new Integer( 1 )); System.out.println(department.getName()); System.out.println(HibernateUtil.getSessionFactory().getStatistics().getEntityFetchCount()); //Prints 1 System.out.println(HibernateUtil.getSessionFactory().getStatistics().getSecondLevelCacheHitCount()); //Prints 2 Output: 1 2 |
Explanation: When another session created from same session factory try to get entity, it is successfully looked up in second level cache and no database call is made.
So now we are clear on how second level cache is used by hibernate.
Download sourcecode
Drop me a comment if any query or suggestion.
Happy Learning !!
No comments:
Post a Comment