Spring bean scopes
The core of spring framework is it’s bean factory and mechanisms to create and manage such beans inside Spring container. The beans in spring container can be created in five scopes.
Lets count them.
- singleton
- prototype
- request
- session
- global-session
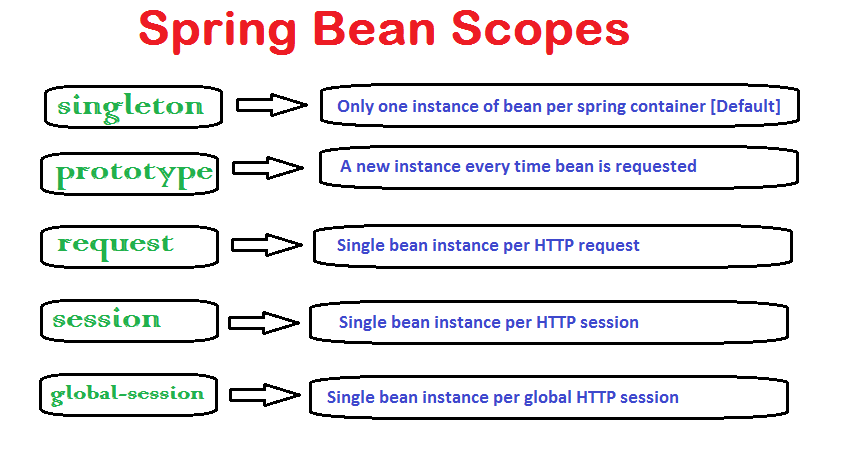
Spring Bean Scopes
All the scope names are self-explanatory but lets make them clear so that there will not be any doubt.
singleton: This bean scope is default and it enforces the container to have only one instance per spring container irrespective of how much time you request for its instance. This singleton behavior is maintained by bean factory itself.
prototype: This bean scope just reverses the behavior of singleton scope and produces a new instance each and every time a bean is requested.
Remaining three bean scopes are web applications related. Essentially these are available through web aware application context (e.g. WebApplicationContext). Global-session is a little different in sense that it is used when application is portlet based. In portlets, there will be many applications inside a big application and a bean with scope of ‘global-session’ will have only one instance for a global user session.
request: With this bean scope, a new bean instance will be created for each web request made by client. As soon as request completes, bean will be out of scope and garbage collected.
session: Just like request scope, this ensures one instance of bean per user session. As soon as user ends its session, bean is out of scope.
global-session:
global-session
is something which is connected to Portlet applications. When your application works in Portlet container it is built of some amount of portlets. Each portlet has its own session, but if your want to store variables global for all portlets in your application than you should store them inglobal-session
. This scope doesn’t have any special effect different from session
scope in Servlet based applications.How to define the bean scope
1) In bean configuration file
1
2
3
4
5
6
7
8
9
| < beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd"> < bean id = "demoBean" class = "com.howtodoinjava.application.web.DemoBean" scope = "session" /> </ beans > |
2) Using annotations
1
2
3
4
5
6
| @Service @Scope ( "session" ) public class DemoBean { //Some code } |
Happy Learning !!
No comments:
Post a Comment