Bubble Sort in Java
Bubble Sort is a generic algorithm to sort the elements and can be implemented in any programming language. The algorithm gets its name from the way smaller elements “bubble” to the top of the list. it is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items and swapping them if they are in the wrong order. This is repeated until the list gets exhausted, which indicates that the list is sorted.
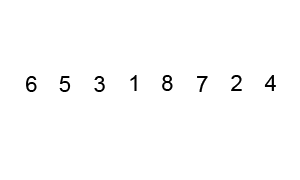
Complexity
Worst Case Complexity O(n²)
Best Case Complexity O(n)
Average Case Complexity O(n²)
Worst case space complexity O(1) auxiliary
Best Case Complexity O(n)
Average Case Complexity O(n²)
Worst case space complexity O(1) auxiliary
Here is an example coded in Java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
| package com.bharat.examples.collections; public class BubbleSortExample { public static void main(String[] args) { int unsortedArray[] = { 20 , 55 , 47 , 0 , - 32 , - 99 , 300 , 7 }; int i; doBubbleSort(unsortedArray); System.out.println( "After sorting, the list elements are: " ); for (i = 0 ; i < unsortedArray.length; i++) { System.out.print(unsortedArray[i] + " " ); } } private static void doBubbleSort( int [] unsortedArray) { int temp; // to hold data while swapping int counter, index; int length = unsortedArray.length; // Loop once for each element in the array. for (counter = 0 ; counter < length - 1 ; counter++) { // Once for each element, minus the counter. for (index = 0 ; index < length - 1 - counter; index++) { // check if we need a swap or not. if (unsortedArray[index] > unsortedArray[index + 1 ]) { // swap temp = unsortedArray[index]; unsortedArray[index] = unsortedArray[index + 1 ]; unsortedArray[index + 1 ] = temp; } } } } } |
Output
1
2
| After sorting, the list elements are: -99 -32 0 7 20 47 55 300 |
No comments:
Post a Comment