Java Thread: notify() and wait() examples
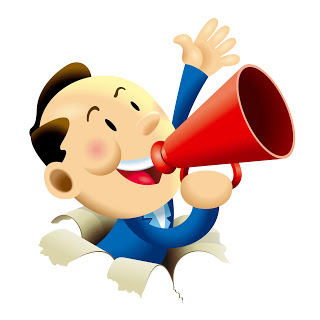
This article contains a code example to demonstrate Java concurrency. They stand for very typical usage. By understanding them, you will have a better understanding about notify() and wait().
0. Some background knowledge
synchronized
keyword is used for exclusive accessing.- To make a method synchronized, simply add the synchronized keyword to its declaration. Then no two invocations of synchronized methods on the same object can interleave with each other.
- Synchronized statements must specify the object that provides the intrinsic lock. When synchronized(this) is used, you have to avoid to synchronizing invocations of other objects’ methods.
wait()
tells the calling thread to give up the monitor and go to sleep until some other thread enters the same monitor and calls notify( ).notify()
wakes up the first thread that calledwait()
on the same object.
1. Notify and wait example 1
In the example above, an object, b, is synchronized. b completes the calculation before Main thread outputs its total value.
Output:
Waiting for b to complete…
Total is: 4950
Waiting for b to complete…
Total is: 4950
If b is not synchonized like the code below:
The result would be 0, 10, etc. Because sum is not finished before it is used.
No comments:
Post a Comment