Java + MongoDB Hello World Example
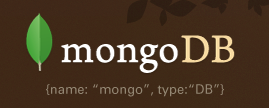
A simple Java + MongoDB hello world example – how to connect, create database, collection and document, save, update, remove, get and display document (data).
Tools and technologies used :
- MongoDB 2.2.3
- MongoDB-Java-Driver 2.10.1
- JDK 1.6
- Maven 3.0.3
- Eclipse 4.2
P.S Maven and Eclipse are both optional, just my personal favorite development tool.
1. Create a Java Project
Create a simple Java project with Maven.
mvn archetype:generate -DgroupId=com.mkyong.core -DartifactId=mongodb -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
2. Get Mongo Java Driver
Download mongo-java driver from github. For Maven users, declares mongo-java driver in
pom.xml
.
pom.xml
...>
org.mongodb
3. Mongo Connection
Connect to MongoDB server. For MongoDB version >= 2.10.0, uses
MongoClient
.// Old version, uses Mongo Mongo mongo = new Mongo("localhost", 27017); // Since 2.10.0, uses MongoClient MongoClient mongo = new MongoClient( "localhost" , 27017 );
If MongoDB in secure mode, authentication is required.
MongoClient mongoClient = new MongoClient(); DB db = mongoClient.getDB("database name"); boolean auth = db.authenticate("username", "password".toCharArray());
4. Mongo Database
Get database. If the database doesn’t exist, MongoDB will create it for you.
DB db = mongo.getDB("database name");
Display all databases.
List<String> dbs = mongo.getDatabaseNames(); for(String db : dbs){ System.out.println(db); }
5. Mongo Collection
Get collection / table.
DB db = mongo.getDB("testdb"); DBCollection table = db.getCollection("user");
Display all collections from selected database.
DB db = mongo.getDB("testdb"); Set<String> tables = db.getCollectionNames(); for(String coll : tables){ System.out.println(coll); }
Note
In RDBMS, collection is equal to table.
In RDBMS, collection is equal to table.
6. Save example
Save a document (data) into a collection (table) named “user”.
DBCollection table = db.getCollection("user"); BasicDBObject document = new BasicDBObject(); document.put("name", "mkyong"); document.put("age", 30); document.put("createdDate", new Date()); table.insert(document);
Refer to this Java MongoDB insert example.
7. Update example
Update a document where “name=mkyong”.
DBCollection table = db.getCollection("user"); BasicDBObject query = new BasicDBObject(); query.put("name", "mkyong"); BasicDBObject newDocument = new BasicDBObject(); newDocument.put("name", "mkyong-updated"); BasicDBObject updateObj = new BasicDBObject(); updateObj.put("$set", newDocument); table.update(query, updateObj);
Refer to this Java MongoDB update example.
8. Find example
Find document where “name=mkyong”, and display it with DBCursor
DBCollection table = db.getCollection("user"); BasicDBObject searchQuery = new BasicDBObject(); searchQuery.put("name", "mkyong"); DBCursor cursor = table.find(searchQuery); while (cursor.hasNext()) { System.out.println(cursor.next()); }
Refer to this Java MongoDB search query example.
9. Delete example
Find document where “name=mkyong”, and delete it.
DBCollection table = db.getCollection("user"); BasicDBObject searchQuery = new BasicDBObject(); searchQuery.put("name", "mkyong"); table.remove(searchQuery);
Refer to this Java MongoDB delete example.
10. Hello World
Let review a complete Java + MongoDB example, see comments for self-explanatory.
App.java
package com.mkyong.core; import java.net.UnknownHostException; import java.util.Date; import com.mongodb.BasicDBObject; import com.mongodb.DB; import com.mongodb.DBCollection; import com.mongodb.DBCursor; import com.mongodb.MongoClient; import com.mongodb.MongoException; /** * Java + MongoDB Hello world Example * */ public class App { public static void main(String[] args) { try { /**** Connect to MongoDB ****/ // Since 2.10.0, uses MongoClient MongoClient mongo = new MongoClient("localhost", 27017); /**** Get database ****/ // if database doesn't exists, MongoDB will create it for you DB db = mongo.getDB("testdb"); /**** Get collection / table from 'testdb' ****/ // if collection doesn't exists, MongoDB will create it for you DBCollection table = db.getCollection("user"); /**** Insert ****/ // create a document to store key and value BasicDBObject document = new BasicDBObject(); document.put("name", "mkyong"); document.put("age", 30); document.put("createdDate", new Date()); table.insert(document); /**** Find and display ****/ BasicDBObject searchQuery = new BasicDBObject(); searchQuery.put("name", "mkyong"); DBCursor cursor = table.find(searchQuery); while (cursor.hasNext()) { System.out.println(cursor.next()); } /**** Update ****/ // search document where name="mkyong" and update it with new values BasicDBObject query = new BasicDBObject(); query.put("name", "mkyong"); BasicDBObject newDocument = new BasicDBObject(); newDocument.put("name", "mkyong-updated"); BasicDBObject updateObj = new BasicDBObject(); updateObj.put("$set", newDocument); table.update(query, updateObj); /**** Find and display ****/ BasicDBObject searchQuery2 = new BasicDBObject().append("name", "mkyong-updated"); DBCursor cursor2 = table.find(searchQuery2); while (cursor2.hasNext()) { System.out.println(cursor2.next()); } /**** Done ****/ System.out.println("Done"); } catch (UnknownHostException e) { e.printStackTrace(); } catch (MongoException e) { e.printStackTrace(); } } }
Output…
{ "_id" : { "$oid" : "51398e6e30044a944cc23e2e"} , "name" : "mkyong" , "age" : 30 , "createdDate" : { "$date" : "2013-03-08T07:08:30.168Z"}} { "_id" : { "$oid" : "51398e6e30044a944cc23e2e"} , "age" : 30 , "createdDate" : { "$date" : "2013-03-08T07:08:30.168Z"} , "name" : "mkyong-updated"} Done
Let use
mongo
console to check the created database “testdb”, collection “user”, and document.$ mongo MongoDB shell version: 2.2.3 connecting to: test > show dbs testdb 0.203125GB > use testdb switched to db testdb > show collections system.indexes user > db.user.find() { "_id" : ObjectId("51398e6e30044a944cc23e2e"), "age" : 30, "createdDate" : ISODate("2013-03-08T07:08:30.168Z"), "name" : "mkyong-updated" }
Download Source Code
Download it – Java-mongodb-hello-world-example.zip (13KB)
3 comments:
How could I initialize mongo-client here using Spring?
How could I initialize mongo-client here using Spring?
Post a Comment