Google App Engine + JSF 2 Example
In this tutorial, we will show you how to develop and deploy a JSF 2.0 web application in Google App Engine (GAE) environment.
Tools and technologies used :
- JDK 1.6
- Eclipse 3.7 + Google Plugin for Eclipse
- Google App Engine Java SDK 1.6.3.1
- JSF 2.1.7
Note
This example is going to reuse this JSF 2.0 hello world example, modify it and merge it with this GAE + Java example.
This example is going to reuse this JSF 2.0 hello world example, modify it and merge it with this GAE + Java example.
1. New Web Application Project
In Eclipse, create a new Web Application project, named as “JSFGoogleAppEngine“.
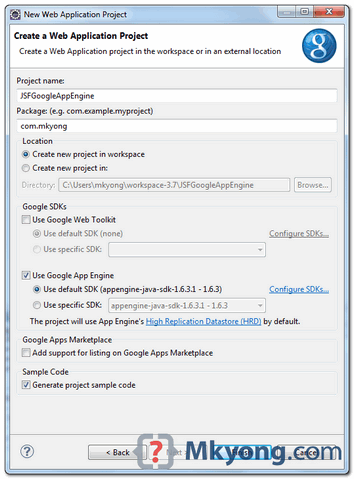
“Google Plugin for Eclipse” will generate a sample of GAE project structure.
2. JSF 2 Dependencies
To use JSF 2 in GAE, you need following jars
- jsf-api-2.1.7.jar
- jsf-impl-2.1.7.jar
- el-ri-1.0.jar
Copy and put it in “war/WEB-INF/lib” folder.
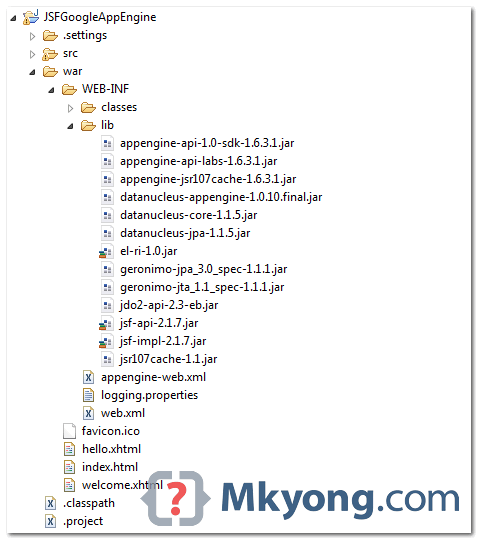
Right click on project folder, select “Properties“. Select “Java Build Path” -> “Libraries” tab, click “Add Jars” button and select above jars.
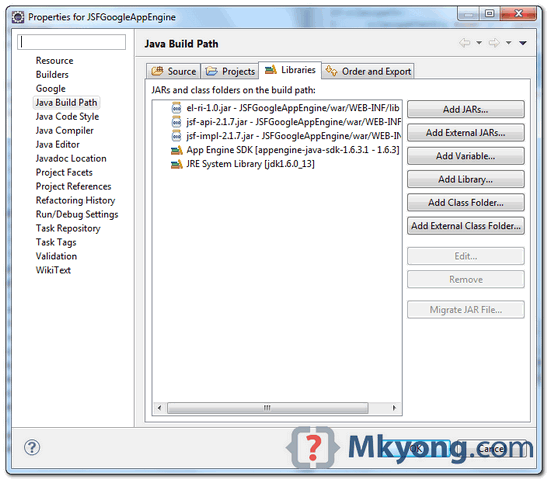
Note
You need to put this
You need to put this
el-ri-1.0.jar
, otherwise, you will hit error message – Unable to instantiate ExpressionFactory ‘com.sun.el.ExpressionFactoryImpl’.3. JSF Managed bean
3.1 Delete plugin generated
JSFGoogleAppEngineServlet.java
, you don’t need this.
3.2 Create a managed bean.
File : src/com/mkyong/HelloBean.java
package com.mkyong; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; import java.io.Serializable; @ManagedBean @SessionScoped public class HelloBean implements Serializable { private static final long serialVersionUID = 1L; private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
3.3 Create a new WebConfiguration.java.
JSF 2 is using “
javax.naming.InitialContext
” that’s not support in GAE.
To solve this, you need to get a copy of the JSF’s source code, clone the
WebConfiguration.java
, comment methods that are using “javax.naming.InitialContext
” class, put it in “src/com/sun/faces/comfig/WebConfiguration.java“. Now, your newly created WebConfiguration.java
class will overload the original WebConfiguration.java
.
Note
Get the full source code of the WebConfiguration.java.
Get the full source code of the WebConfiguration.java.
I don’t think GAE team will white-list this jar, just hope JSF’s team can fix this in future release.
4. JSF Pages
4.1 Create
hello.xhtml
page, accept a user input and pass it to helloBean.
File : war/hello.xhtml
version="1.0" encoding="UTF-8"?>
4.2 Create
welcome.xhtml
page, display the user input from hellobean.
File : war/welcome.xhtml
version="1.0" encoding="UTF-8"?>
4.3 Delete the plugin generated
index.html
file, you don’t need this.5. web.xml
Update web.xml, integrate JSF 2.
File : web.xml
version="1.0" encoding="utf-8"?>
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5">
JavaServerFaces
Note
GAE do not support server side state management, so, you need to define “
GAE do not support server side state management, so, you need to define “
javax.faces.STATE_SAVING_METHOD
” to “client
“, to avoid of this “View /hello.xhtml could not be restored” error message in GAE production environment.6. Enable Session in GAE
Update
File : appengine-web.xml
appengine-web.xml
, enable session support, JSF need this.File : appengine-web.xml
version="1.0" encoding="utf-8"?>
xmlns="http://appengine.google.com/ns/1.0">
7. Directory Structure
Review final directory structure.
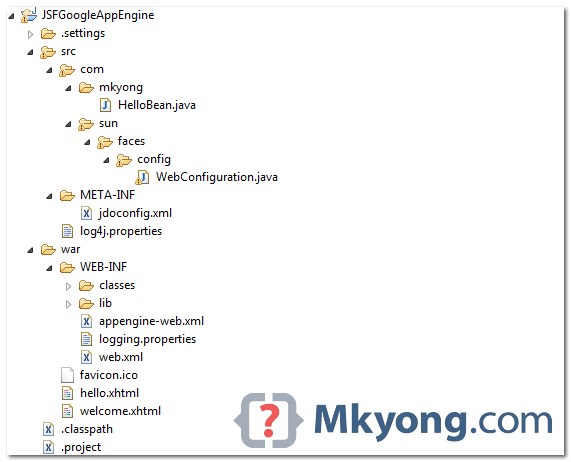
8. Run on Local
Right click on the project, run as “Web Application”.
URL : http://localhost:8888/hello.jsf
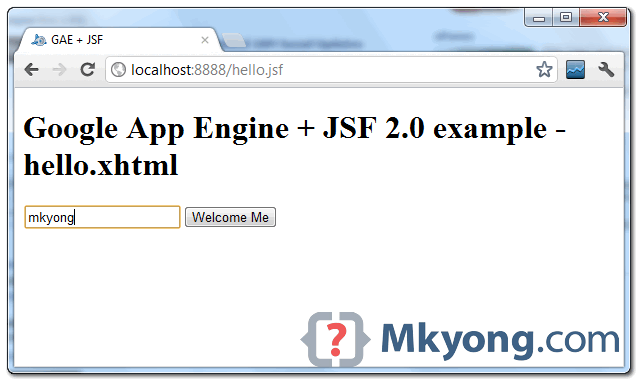
Click on the button.
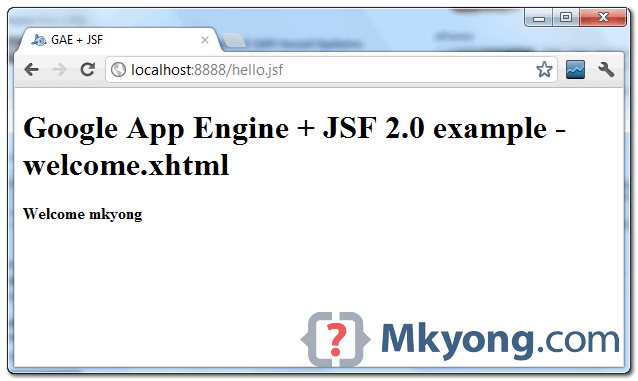
10. Deploy on GAE
Update
appengine-web.xml
file, add your App Engine application ID.
File : appengine-web.xml
version="1.0" encoding="utf-8"?>
xmlns="http://appengine.google.com/ns/1.0">
mkyong-jsf2gae
Select project, click on Google icon, “Deploy to App Engine“.
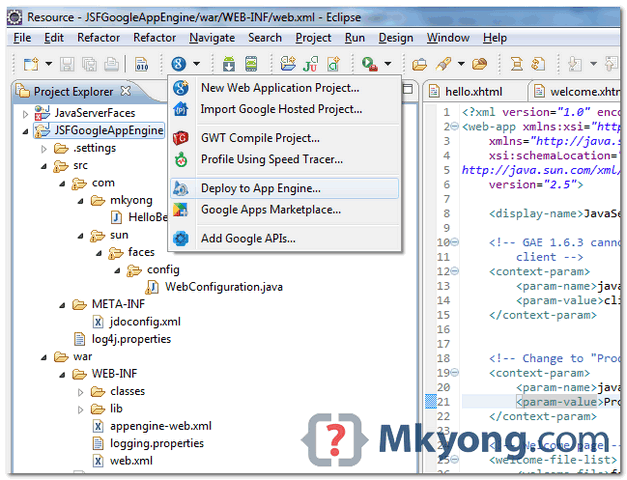
URL : http://mkyong-jsf2gae.appspot.com/hello.jsf
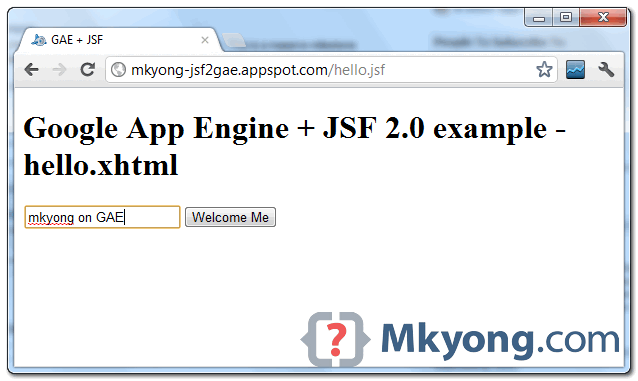
Download Source Code
Due to large file size, all JSF and GAE jars are excluded.
Download – JSF2-GoogleAppEngine-Example.zip (42 KB)
1 comment:
yeezy boost 350
michael kors purses
vans shoes
retro jordans
christian louboutin shoes
supreme new york
golden goose sneakers
nike air max 270
michael kors outlet
off white jordan 1
Post a Comment