How To Create A Java Project With Maven
In this tutorial, we will show you how to use Maven to create a single Java project, make it support Eclipse IDE, and packaging into a “jar” file.
Tools used :
- Maven 3.0.5
- Eclipse 4.2
- JDK 6
1. Create a Project from Maven Template
In a terminal (*uix or Mac) or command prompt (Windows), navigate to the folder you want to store the project. Issue following commands :
mvn archetype:generate -DgroupId={project-packaging} -DartifactId={project-name} -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This tell Maven to create a Java project from “maven-archetype-quickstart” template. If you ignore the
archetypeArtifactId
argument, a list of the templates will be listed for you to choose.
For example,
$ mvn archetype:generate -DgroupId=com.mkyong -DartifactId=NumberGenerator -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false [INFO] Scanning for projects... [INFO] [INFO] -- omitted for readability [INFO] ---------------------------------------------------------------------------- [INFO] Using following parameters for creating project from Old (1.x) Archetype: maven-archetype-quickstart:1.0 [INFO] ---------------------------------------------------------------------------- [INFO] Parameter: groupId, Value: com.mkyong [INFO] Parameter: packageName, Value: com.mkyong [INFO] Parameter: package, Value: com.mkyong [INFO] Parameter: artifactId, Value: NumberGenerator [INFO] Parameter: basedir, Value: /Users/mkyong/Documents/workspace [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] project created from Old (1.x) Archetype in dir: /Users/mkyong/Documents/workspace/NumberGenerator [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 3.917s [INFO] Finished at: Mon Dec 17 18:53:58 MYT 2012 [INFO] Final Memory: 9M/24M [INFO] ------------------------------------------------------------------------
In above case, a new Java project named “NumberGenerator”, and the entire project directory structure is created automatically.
2. Maven Directory Layout
Maven created following standard directory layout. Please check this official guide to understand more.
NumberGenerator |-src |---main |-----java |-------com |---------mkyong |-----------App.java |---test |-----java |-------com |---------mkyong |-----------AppTest.java |-pom.xml
In simple, all source code puts in folder
/src/main/java/project-package
, all unit test code puts in/src/test/java/project-package
.
And yes, a standard
pom.xml
is generated. This POM file is like the Ant build.xml
file, it stated the entire project information, everything from directory structure, project plugins to project dependencies… read this official POM guide.
pom.xml
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
4.0.0
3. Works with Eclipse IDE
To convert Maven project to support Eclipse IDE, in terminal, navigate to “NumberGenerator” project, issue this command :
mvn eclipse:eclipse
It will generate all project files that are required by Eclipse IDE.
Figure : Imports it into Eclipse IDE
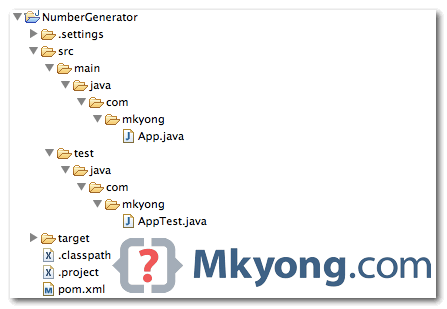
4. Update POM
The default
pom.xml
is too simple, often times you need to add the compiler plugin to tell Maven which JDK version to compile your project. (The default JDK1.4 is too old).org.apache.maven.plugins
Update the jUnit from 3.8.1 to latest 4.11.
junit
Maven Coordinate
Above XML code snippet is called “Maven Coordinate”, If you want junit jar, you need to find out its corresponding Maven coordinate. It applied to all other dependencies, such as Spring, Hibernate, Apache common and etc, just goMaven center repository and find out what is the correct Maven coordinate of the dependency.
Above XML code snippet is called “Maven Coordinate”, If you want junit jar, you need to find out its corresponding Maven coordinate. It applied to all other dependencies, such as Spring, Hibernate, Apache common and etc, just goMaven center repository and find out what is the correct Maven coordinate of the dependency.
pom.xml – full version.
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
4.0.0
In terminal, issue the same command
mvn eclipse:eclipse
again, Maven will download the plugins and project dependencies and store it into your local Maven repository automatically.5. Update Business Logic
Test driven development (TDD), update the unit test first, to make sure
App
object has a method to generate a unique key that contains exactly 36 alphabets.
AppTest.java
package com.mkyong; import org.junit.Assert; import org.junit.Test; public class AppTest { @Test public void testLengthOfTheUniqueKey() { App obj = new App(); Assert.assertEquals(36, obj.generateUniqueKey().length()); } }
Complete the business logic.
App.java
package com.mkyong; import java.util.UUID; /** * Generate a unique number * */ public class App { public static void main( String[] args ) { App obj = new App(); System.out.println("Unique ID : " + obj.generateUniqueKey()); } public String generateUniqueKey(){ String id = UUID.randomUUID().toString(); return id; } }
6. Maven Packaging
Now, we will use Maven to compile this project and output to a “jar” file. Refer to the
pom.xml
file, the packaging
element defined what is the packaging format or output.
pom.xml – full version.
...>
4.0.0
In terminal, issue
mvn package
:$pwd /Users/mkyong/Documents/workspace/NumberGenerator $ mvn package [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building NumberGenerator 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] ...omitted for readability ------------------------------------------------------- T E S T S ------------------------------------------------------- Running com.mkyong.AppTest Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.078 sec Results : Tests run: 1, Failures: 0, Errors: 0, Skipped: 0 [INFO] [INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ NumberGenerator --- [INFO] Building jar: /Users/mkyong/Documents/workspace/NumberGenerator/target/NumberGenerator-1.0-SNAPSHOT.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 3.064s [INFO] Finished at: Mon Dec 17 23:38:05 MYT 2012 [INFO] Final Memory: 15M/135M [INFO] ------------------------------------------------------------------------
It compiles, run your unit test and generate the project “jar” file in
project/target
folder.
Figure : Final project directory structure.
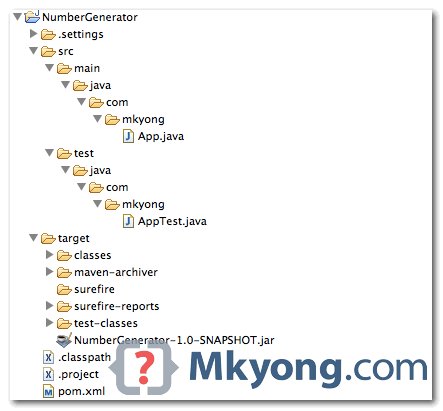
7. Demo
Run the generated jar file ~
$pwd /Users/mkyong/Documents/workspace/NumberGenerator $ java -cp target/NumberGenerator-1.0-SNAPSHOT.jar com.mkyong.App Unique ID : f1947107-2deb-4926-a635-ea3db61453e8 $ java -cp target/NumberGenerator-1.0-SNAPSHOT.jar com.mkyong.App Unique ID : 98ed9e1c-d1bc-47f1-847d-64db451ce0ff
Download Source Code
Download it – Maven-NumberGenerator.zip(12 KB)
No comments:
Post a Comment