Spring Data MongoDB Hello World Example
In this tutorial, we show you how to use “SpringData for MongoDB” framework, to perform CRUD operations in MongoDB, via Spring’s annotation and XML schema.
Updated on 1/04/2013
Article is updated to use latest SpringData v 1.2.0.RELEASE, it was v1.0.0.M2.
Article is updated to use latest SpringData v 1.2.0.RELEASE, it was v1.0.0.M2.
Tools and technologies used :
- Spring Data MongoDB – 1.2.0.RELEASE
- Spring Core – 3.2.2.RELEASE
- Java Mongo Driver – 2.11.0
- Eclipse – 4.2
- JDK – 1.6
- Maven – 3.0.3
P.S Spring Data requires JDK 6.0 and above, and Spring Framework 3.0.x and above.
1. Project Structure
A classic Maven’s style Java project directory structure.
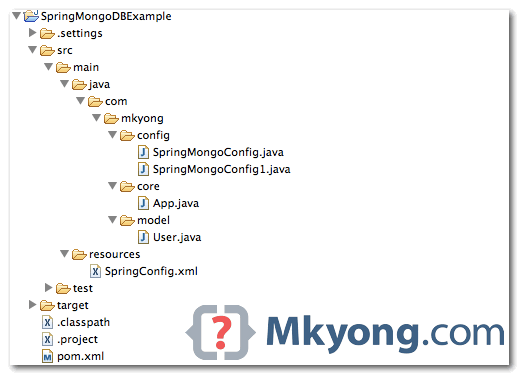
2. Dependency
The following libraries are required :
Currently, the “
spring-data-mongodb
” jar is only available in “http://maven.springframework.org/milestone“, so, you have to declare this repository also.
Updated on 13/09/2012
spring-data-mongodb
is available at the Maven central repository, Spring repository is no longer required.
pom.xml
xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/maven-v4_0_0.xsd">
4.0.0
3. Spring Configuration, Annotation and XML
Here, we show you two ways to configure Spring data and connect to MongoDB, via annotation and XML schema.
Note
Refer to this official reference Connecting to MongoDB with Spring.
Refer to this official reference Connecting to MongoDB with Spring.
3.1 Annotation
Extends the
Extends the
AbstractMongoConfiguration
is the fastest way, it helps to configure everything you need to start, likemongoTemplate
object.
SpringMongoConfig.java
package com.mkyong.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.mongodb.config.AbstractMongoConfiguration; import com.mongodb.Mongo; import com.mongodb.MongoClient; @Configuration public class SpringMongoConfig extends AbstractMongoConfiguration { @Override public String getDatabaseName() { return "yourdb"; } @Override @Bean public Mongo mongo() throws Exception { return new MongoClient("127.0.0.1"); } }
Alternatively, I prefer this one, more flexible to configure everything.
SpringMongoConfig1.java
package com.mkyong.config; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.mongodb.MongoDbFactory; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.data.mongodb.core.SimpleMongoDbFactory; import com.mongodb.MongoClient; @Configuration public class SpringMongoConfig1 { public @Bean MongoDbFactory mongoDbFactory() throws Exception { return new SimpleMongoDbFactory(new MongoClient(), "yourdb"); } public @Bean MongoTemplate mongoTemplate() throws Exception { MongoTemplate mongoTemplate = new MongoTemplate(mongoDbFactory()); return mongoTemplate; } }
And load it with
AnnotationConfigApplicationContext
:ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringMongoConfig.class); MongoOperations mongoOperation = (MongoOperations)ctx.getBean("mongoTemplate");
3.2 XML Schema
SpringConfig.xml
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mongo="http://www.springframework.org/schema/data/mongo"
xsi:schemaLocation="http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/data/mongo
http://www.springframework.org/schema/data/mongo/spring-mongo-1.0.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
host="127.0.0.1" port="27017" />
dbname="yourdb" />
id="mongoTemplate" class="org.springframework.data.mongodb.core.MongoTemplate">
name="mongoDbFactory" ref="mongoDbFactory" />
And include it with Spring’s
GenericXmlApplicationContext
:ApplicationContext ctx = new GenericXmlApplicationContext("SpringConfig.xml"); MongoOperations mongoOperation = (MongoOperations)ctx.getBean("mongoTemplate");
So, XML or Annotation?
Actually, both are doing the same thing, it’s just based on personal preferences. Personally, I like XML to configure things.
Actually, both are doing the same thing, it’s just based on personal preferences. Personally, I like XML to configure things.
4. User Model
An User object, annotated @Document – which collection to save. Later, we show you how to use Spring data to bind this object to / from MongoDB.
User.java
package com.mkyong.model; import org.springframework.data.annotation.Id; import org.springframework.data.mongodb.core.mapping.Document; @Document(collection = "users") public class User { @Id private String id; String username; String password; //getter, setter, toString, Constructors }
5. Demo – CRUD Operations
Full example to show you how to use Spring data to perform CRUD operations in MongoDB. The Spring data APIs are quite clean and should be self-explanatory.
App.java
package com.mkyong.core; import java.util.List; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.data.mongodb.core.MongoOperations; import org.springframework.data.mongodb.core.query.Criteria; import org.springframework.data.mongodb.core.query.Query; import org.springframework.data.mongodb.core.query.Update; import com.mkyong.config.SpringMongoConfig; import com.mkyong.model.User; //import org.springframework.context.support.GenericXmlApplicationContext; public class App { public static void main(String[] args) { // For XML //ApplicationContext ctx = new GenericXmlApplicationContext("SpringConfig.xml"); // For Annotation ApplicationContext ctx = new AnnotationConfigApplicationContext(SpringMongoConfig.class); MongoOperations mongoOperation = (MongoOperations) ctx.getBean("mongoTemplate"); User user = new User("mkyong", "password123"); // save mongoOperation.save(user); // now user object got the created id. System.out.println("1. user : " + user); // query to search user Query searchUserQuery = new Query(Criteria.where("username").is("mkyong")); // find the saved user again. User savedUser = mongoOperation.findOne(searchUserQuery, User.class); System.out.println("2. find - savedUser : " + savedUser); // update password mongoOperation.updateFirst(searchUserQuery, Update.update("password", "new password"),User.class); // find the updated user object User updatedUser = mongoOperation.findOne(searchUserQuery, User.class); System.out.println("3. updatedUser : " + updatedUser); // delete mongoOperation.remove(searchUserQuery, User.class); // List, it should be empty now. List<User> listUser = mongoOperation.findAll(User.class); System.out.println("4. Number of user = " + listUser.size()); } }
Output
1. user : User [id=516627653004953049d9ddf0, username=mkyong, password=password123] 2. find - savedUser : User [id=516627653004953049d9ddf0, username=mkyong, password=password123] 3. updatedUser : User [id=516627653004953049d9ddf0, username=mkyong, password=new password] 4. Number of user = 0
Download Source Code
Download it – SpringMongoDB-HelloWorld-Example.zip (24 KB)
No comments:
Post a Comment